숫자 변환하기 도움말
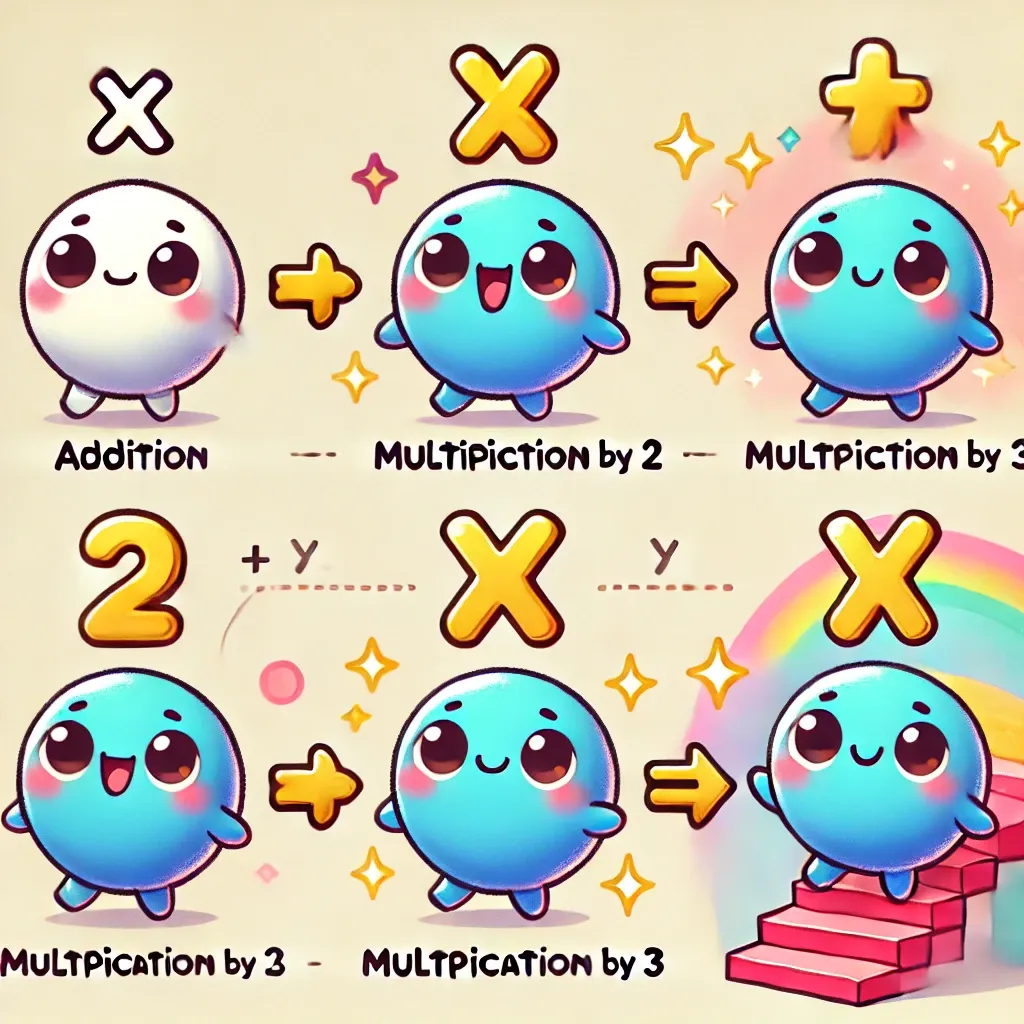
자연수 x
를 y
로 변환하려고 합니다. 사용할 수 있는 연산은 다음과 같습니다.
x
에n
을 더합니다x
에 2를 곱합니다.x
에 3을 곱합니다.
자연수 x
, y
, n
이 매개변수로 주어질 때, x
를 y
로 변환하기 위해 필요한 최소 연산 횟수를 return하도록 solution 함수를 완성해주세요. 이때 x
를 y
로 만들 수 없다면 -1을 return 해주세요.
https://school.programmers.co.kr/learn/courses/30/lessons/154538
import java.util.*;
public class Main21 {
public static void main(String[] args) {
int x = 2;
int y = 5;
int z = 4;
var reuslt = solution(x, y, z);
System.out.println(reuslt);
}
public static int solution(int x, int y, int n) {
Queue<Integer> queue1 = new LinkedList<>();
Queue<Integer> queue2 = new LinkedList<>();
queue1.add(y);
int tryCount = 0;
while (true) {
var targetQueue = queue1.isEmpty() ? queue2 : queue1;
var otherQueue = !queue1.isEmpty() ? queue2 : queue1;
if (targetQueue.contains(x)) {
return tryCount;
}
if (targetQueue.isEmpty()) {
return -1;
}
if (Collections.max(targetQueue) < x) {
return -1;
}
while (!targetQueue.isEmpty()) {
var temp = targetQueue.poll();
if (temp < x) {
continue;
}
if (temp % 2 == 0 && temp / 2 >= x) {
otherQueue.add(temp / 2);
}
if (temp % 3 == 0 && temp / 3 >= x) {
otherQueue.add(temp / 3);
}
if (temp - n >= x) {
otherQueue.add(temp - n);
}
}
tryCount++;
}
}
}